file_get_contents() wrapper enables accessing URL objects the same way as local files.
You can check if the file_get_contents() wrapper is enabled in your active PHP version by creating and checking phpinfo file:
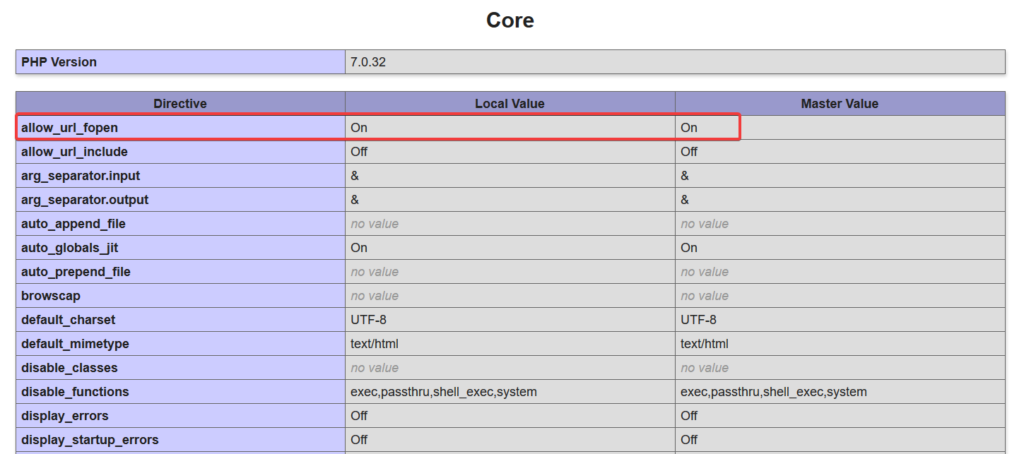
or a simple PHP code like this:
<?php
if( ini_get('allow_url_fopen') ) {
die('allow_url_fopen is enabled.');
} else {
die('allow_url_fopen is disabled.');
}
?>
If allow_url_fopen isn’t enabled in your PHP version, but your application uses it, you will see the following Warning in your error_log file:
Warning: file_get_contents(): http:// wrapper is disabled in the server configuration by allow_url_fopen=0
Here are a few solutions to this issue:
Enable allow_url_fopen in php.ini
allow_url_fopen is disabled on most shared hosting for security reasons, so if you don’t have access to php.ini file directly, you should ask your hosting provider to enable -> allow_url_fopen by turning on the following values in php.ini file:
allow_url_fopen = 1
allow_url_include = 1
On cPanel you can modify php.ini file either from MultiPHP or PHP Selector option on CloudLinux:
To enable allow_url_fopen on PHPSelector in CloudLinux, from cPanel navigate to PHP Selector > Options and check the allow_url_fopen option:
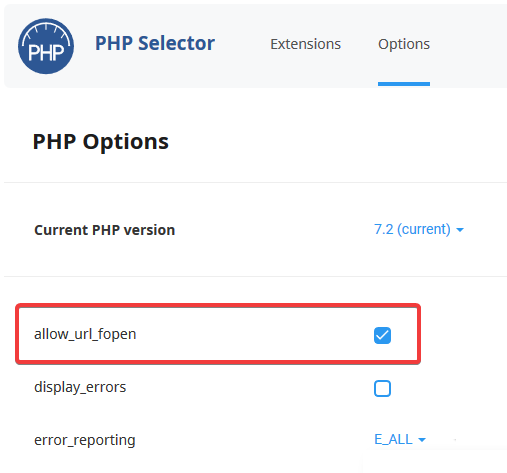
To enable allow_url_fopen on MultiPHP INI Editor in cPanelr, navigate to MultiPHP INI Editor > Basic Mode select your domain name and the enable the allow_url_fopen option:
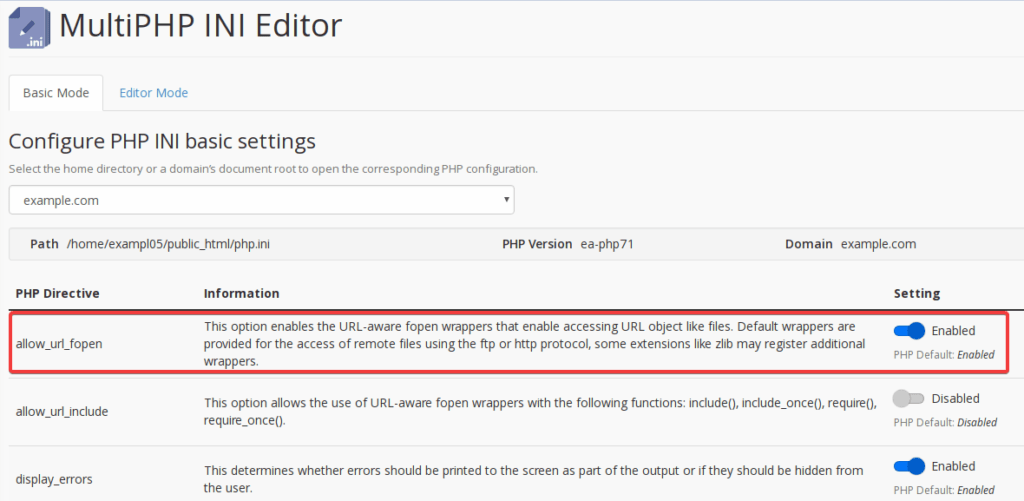
For LiteSpeed webservers, you will also have to restart php processes to take effect:
pkill lsphp
Replace file_get_contents
calls in your code with CURL
Here is a simple CURL method from Tushar that behaves exactly the same way as file_get_contents:
function curl_get_file_contents($URL)
{
$c = curl_init();
curl_setopt($c, CURLOPT_RETURNTRANSFER, 1);
curl_setopt($c, CURLOPT_URL, $URL);
$contents = curl_exec($c);
curl_close($c);
if ($contents) return $contents;
else return FALSE;
}
Source: stackoverflow.com