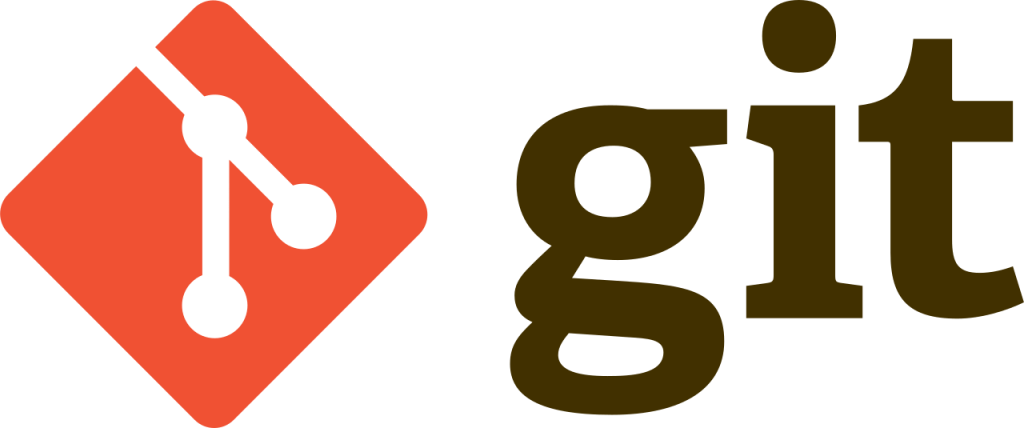
This is a practical git tutorial for beginner, focus on the commands that are used daily by every programmer.
Related:
Git Glossary
Want to learn Git? Check out this quick summary of the most important terms and commands.
- git: an open source, distributed version-control system
- commit: a Git object, a snapshot of your entire repository compressed into a SHA
- branch: a lightweight movable pointer to a commit
- master: default name for the first branch
- clone: a local version of a repository, including all commits and branches
- remote: a common repository on GitHub that all team members use to exchange their changes
- fork: a copy of a repository on GitHub owned by a different user
- pull request: a place to compare and discuss the differences introduced on a branch with reviews, comments, integrated tests, and more
- HEAD: representing your current working directory
- merge: joining two or more commit histories
Install Git
For Windows you can download git from here, on Mac you can use Homebrew, and here is how to install git on Linux:
Windows
Download and install the latest Git for Windows Installer here.
Mac
Download and install the latest Git for Mac installer here.
Linux
sudo add-apt-repository ppa:git-core/ppa
sudo apt-get update
sudo apt-get install git
Auto update to latest git
sudo add-apt-repository ppa:git-core/ppa
sudo apt-get update
sudo apt-get upgrade
Install on Centos/Redhat
sudo yum install git
Install on Fedora
sudo dnf install git
Install on Arch Linux
sudo pacman -Sy git
Install on Gentoo
sudo emerge --ask --verbose dev-vcs/git
Configure Git
Configure user information for all local repositories, replace highlighted details with your actual details.
These details will be associated with any commits that you create.
git config --global user.name "Stefan Pejcic"
Setup default name
git config --global user.email "stefan@pcx3.com"
Setup default email
You can also associate a name to the remote server address, to avoid typing it every time when you push changes to the server.
git remote add gh https://github.com/stefanpejcic/stefan_init.git
New Project
To create a new project in git we either create a brand new project or clone an existing one. When a repository was initialized locally, you have to push it to GitHub afterwards.
git init
Create a git repository in current directory.
git clone url_or_path
Clone the repository from url_or_path to current directory.
git clone url_or_path
Clone the repository from url_or_path to current directory.
git remote -v
Check remote Git URL.
Commit Files
After you made changes to the file, then you can commit your changes to local repository.
git status
Show current status – list all modified files
git add .
Add changes from current directory to local staging area
Commit changes to local repository with a message
git commit -m"message"
Fix last commit message
git commit --amend -m "new commit message"
Reset to last commit, but keep your changes
git reset --soft HEAD^
Reset to last commit, and throw away all your changes
git reset --hard HEAD^
Examples
git add index.cfm get.cfc
git commit -m"Fixed bug 123."
Commit several files from the directory
git add .
git commit -m"Fixed bug 123."
Commit all files in current directory
git add -A .
git commit -m"Fixed bug 123."
Commit all NEW files in current directory
git log
We can also view the commit logs before pushing them to a remote repository using git log command.
Sync with Remote Repository
With Push and Pull you can sync files between your local repository and the remote one.
git pull
Pull files from remote repository to your local one
git push
Push files from local repository to the remote one
Examples
Update your local repository (and working directory) from remote server
git pull https://github.com/stefanpejcic/python.git
You can also force a pull from the server by removing all local changes
git reset --hard
git clean -xdf
git pull remote_server_or_name
You can also request a clean sync (a fresh copy) of a remote repository
git remote add upstream https://github.com/stefanpejcic/python
git fetch upstream
git checkout master
git reset --hard upstream/master
git push origin master --force
Remove / Delete Files
With reset / rm commands you can unadd a file from the staging area or delete it entirely from the remote repository.
Unadd a specific file from the staging area
git reset myFileName
Unadd ALL files from the staging area
git reset .
Discard ALL local changes
git reset --hard
Delete a specific file (or files) from the local repository
git rm file_name
Delete an entire directory from the local repository
git rm -r dir_name
delete all untracked local files and directories (DANGEROUS)
git clean -xdf
Find Difference
Here’s how to find differences between various areas of git on your local repository: working directory, staging area and HEAD.
Find out when is a line added to a file
git blame file_name
Show state of last commit, staging area and working directory
git status
Get commit IDs
git log file_name
Show difference between last changes
git log -p -2 --color
Get last 3 commit IDs
git log -3
Show ALL difference between working directory & index
git diff --color
If you see no difference, then check staging
git diff --staged
Show difference for 1 file between working directory & index
git diff --color filename
Show difference between index & latest commit (HEAD)
git diff --color --staged ‹commitID›
Show difference between last commit & working directory
git diff --color ‹commitID›
Revert Change
You can revert changes in the working directory all the way up to the last commit
Update the file_name in working directory
git checkout -- file_name
update the dir_full_path to the commit version in HEAD
git checkout HEAD -- dir_full_path
Revert file to last commit
git checkout HEAD -- file_name
Revert file to the commit before last commit
git checkout HEAD^ -- file_name
Remove ALL untracked files
git clean -d -x -f
- -d → Delete untracked directories
- -x → ignore the .gitignore file. Delete them.
- -f → force it
Git Branching
Use git branch to list all local branches, the current branch is indicated by a asterisk *
Show all local branches
git branch
- -r → Show all remote branches
- -a → Show all local and remote branches
Show branches and their commits
git show-branch
Create a new branch name from “master”
git branch name master
Switch to a branch by updating files in the current directory
git checkout branch_name
Clone a specific branch (without fetching other branches)
git clone --b branchname --single-branch remote-repo-url
Clone a specific branch (still fetched all files from each branch)
git clone --branch branchname remote-repo-ur
Rename a branch
git branch -m old_name newName
Rename a branch even if a branch with the same name exists
git branch -M old_name newName
Fetch all remote branches
git fetch -- all
Merge a branch named name into the current branch
git merge name
Delete a branch (must be merged first)
git branch -d branch_name
Force delete a branch (even if not merged)
git branch -D branch_name
As you can see above, we have 2 different arguments, one with small case d and one with capital case D.
- -d option stands for –delete
- -D option stands for –delete –force
Delete a remote branch
git push {remote_name} –delete {branch_name}
Git Archive
git archive lets you create a copy of your git directory, without the .git data, in zip or tar.gz format.
Archive all files from the current directory to myArchive file
git archive -o ../myArchive.zip HEAD
- .tar HEAD → use tar
- .tar.gz HEAD → use tar.gz
- .tar.xz HEAD → use tar.xz
zip -d xyz some.zip
Unzip content to a new parent directory xyz
Git Stash
Save working directory (all uncommitted changes) in a temp storage
Save working directory into temp storage
git stash save
Restore working directory from temp storage
git stash pop
List your stash
git stash list
Git Logs
The Git Log tool allows you to view information about previous commits that have occurred in a project.
Commits are shown in reverse chronological order (the most recent commits first).
Force the log tool to display all commits
git log
-all will display all commits
-p option will display diff of changes for each commit
-n display a specified number of recent commitsFilter commits by author or committer
git log --author <name>
git log --committer <name>
Filter commits by X days ago
git log --before <date>
git log --after <date>
Filter commits by date range
git log --after <date> --before <date>
You can also format the Git log output
git log --pretty=format:"Commit Hash: %H, Author: %aN, Date: %aD"
Git Ignore File
Sometimes it may be a good idea to exclude files from being tracked with Git. This is typically done in a special file named .gitignore. You can find helpful templates for .gitignore files at github.com/github/gitignore.
Example of .gitignore file
~/git/HiddenProject
.git
.gitignore
README.md
HiddenProject.py
.gitignore file is located in the top level directory and contains file name patterns that git should ignore.
We need your help!
Do you know a git command that we haven’t included in this git CheatSheet?
Help us keep the git CheatSheet up-to-date and enrich it by sharing the useful git commands that you know with other system administrators.