In this article, I’m going to talk about the usage of Apache Tomcat’s shutdown functionality and how one can use it to remotely shutdown multiple Tomcat applications.
Apache Tomcat has a shutdown functionality that allows you to programmatically shutdown a Tomcat server regardless of which operating system it is running on. This basically means that you can shut down multiple Tomcat instances in the same way on Linux and Windows servers.
During the Tomcat boot-up process, the configuration files are loaded, and the most important configuration file among them is the server.xml file. This config file contains the shutdown port and the command to shutdown Tomcat.
Default Tomcat shutdown port is 8005 and the command is SHUTDOWN
<Server port="8005" shutdown="SHUTDOWN">
Changing the Tomcat shutdown port
These can be defined in the server.xml file, and I recommend that on a production server you either change the shutdown command/port or enable its usage from the localhost only.
After changing the default port/command, if someone tries to shutdown Tomcat using the default port/command will get the following error message:
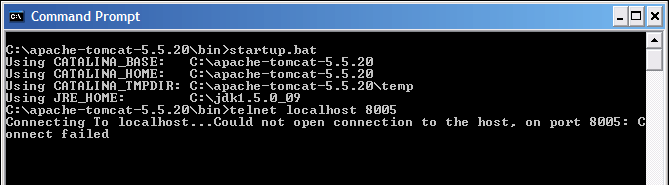
How to shutdown Tomcat remotely
All that one has to do shutdown tomcat is to know the following.
– Host machine where the Tomcat is running.
– Shutdown port of Tomcat. {Default : 8005}
– Command to shutdown Tomcat. {Default : “SHUTDOWN”}
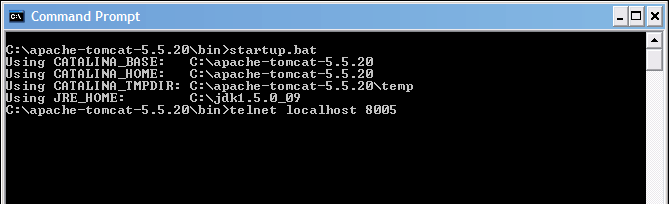
The easy way to shutdown a Tomcat server is to just telnet the server with the shutdown port, for example:
telnet localhost 8005
Another way is to write a client application using java.net.Socket class, connect to the shutdown port and send the command to shutdown. Below you have a code snippet to shutdown Tomcat from remote:
public void shutDownTomcat() {
InetAddress serverAddress = null;
int serverPortNum = 8005;
Socket socket = null;
String magicWord = "SHUTDOWN";
byte[] buffer = null;
OutputStream os = null;
try {
/*
* Change to InetAddress.getHostByName(somehostname) if client is
* running from a remote machine
*/
serverAddress = InetAddress.getLocalHost();
socket = new Socket(serverAddress, serverPortNum);
os = socket.getOutputStream();
buffer = magicWord.getBytes();
os.write(buffer, 0, buffer.length);
os.flush();
} catch (UnknownHostException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} finally {
if (os != null) {
try {
os.close();
} catch (IOException e) {
e.printStackTrace();
}
os = null;
}
}
}
NOTE: The above approach should be taken with caution because it is also possible to shutdown all Tomcat instances within a network by scanning for all hosts.