Here is a simple PHP script that you can use to test if a port is open on the server or not.
<?php
$host = $_SERVER['SERVER_ADDR'];
$ports = array(21, 25, 80, 81, 110, 443, 3306);
foreach ($ports as $port)
{
$connection = @fsockopen($host, $port);
if (is_resource($connection))
{
echo '<h2>' . $host . ':' . $port . ' ' . '(' . getservbyport($port, 'tcp') . ') is open.</h2>' . "\n";
fclose($connection);
}
else
{
echo '<h2>' . $host . ':' . $port . ' is not responding.</h2>' . "\n";
}
}
?>
And if you want to check for open ports on another server, get it’s IP address and put it instead of the $_SERVER[‘SERVER_ADDR’]; like this:
$host = $_SERVER['SERVER_ADDR'];
$host = '142.250.190.46';
Create a new file e.g. port-check.php and put the above snippet inside then open it in your browser. that’s it.
The output will be similar to this:
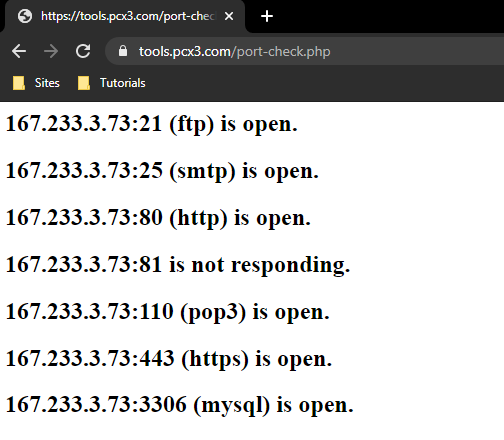
Ok how to open the 81 if was not open?
Hi there, to open a specific port on a server you need root-level access.
The procedure to open a port on the firewall is different depending on your OS and firewall installed (iptables, csf, apf, ferm..) https://pcx3.com/?s=port